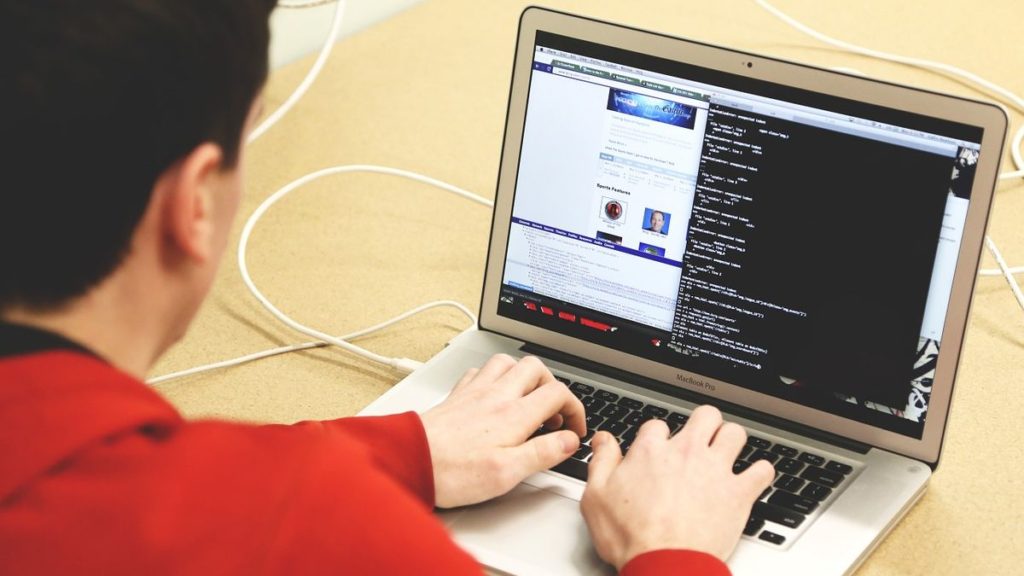
Interviewing for a programming job can be a very stressful experience. There is no way to predict what questions will be asked of you. C# programming interview questions are even more challenging because it is a very versatile language with a lot of areas to learn.
What do you do if you don’t know the answer to a question? How do you go about landing the job of a lifetime? This article, written by a professional programmer, will help guide you.
The purpose of programming interview questions
Programing interview questions have their place. However, you need to remember that they are only a small part of a larger interview process.
A potential employer wants to know what you are skilled at and how you can best benefit their team. They also know that you’re human and that you can’t be expected to know everything. On the job, you’ll be expected to research solutions online instead of doing everything from memory.
Because of this, programming interview questions are designed to get a glimpse into your development thought process and confirm that you are capable of writing code.
How difficult are C# programming interview questions?
The difficulty of the questions you will be asked depend on the role you are applying for. Entry level positions will have very few questions specific to any given language because you aren’t expected to be an expert.
As you move up in your career, you can expect the questions to become more challenging. For example, for a Technical Lead position you may even be expected to code a solution to a non-trivial problem during or prior to the interview and be asked to explain your thought process and design choices.
This is why in order to continue to grow in your career you will want to gain a lot of professional experience and practice solving interview-style programming questions.
Programming interview mindset
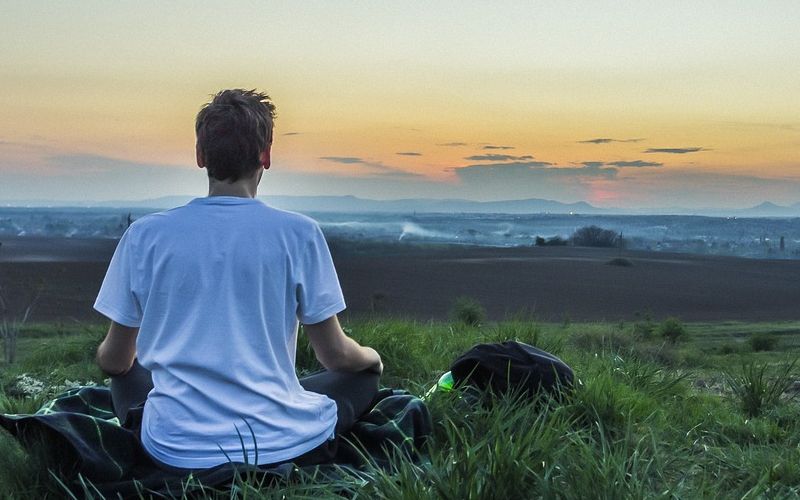
Programming interviews require you to practice a lot before you attend them or you run the risk of failing the interview when your nerves get the best of you.
During my first interview applying for a developer job I became so nervous that I began to give non-sensical answers. It could have been a disaster but I had the presence of mind to let the interviewer know that I was becoming overwhelmed and was able to pause and resume after a minute. Because I was able to recover from that error, I was able to get the job.
It is this lesson that has taught me how important it is to mentally prepare for interviews…and especially more so for high pressure programming interviews where I’m expected to produce code on demand.
Here are some things you can do to reduce the stress of a programing interview and help you to land that job.
Learn to relax while under pressure during interviews
Everyone feels nervous when their livelihood is on the line. However, these emotions can get in your way if you don’t get them under control. I’ve almost lost jobs when I’ve let my emotions get in my way. I’ve also aced interviews when I went into them with an “I don’t care if I get this job” mindset.
Before you set foot into the interviewing crucible, take some time to learn how to relax under pressure. It will make a world of difference and allow you to ace any programming interview.
Here are a few ways that can make a massive impact:
- Meditation – Learn how to return to a place of calm by focusing on your breathing. Even 10 minutes of focused practice can give you an edge.
- Visualization – Mentally walk through the entire interview process and how you will respond. This includes going over previous interviews and refining your responses until they flow naturally.
- Practice interviewing with a friend – If you have a developer friend who would be willing to give you a hand, have them ask you C# programming interview questions to test your knowledge. You’ll be able to gain valuable feedback and test your knowledge at the same time.
- Practice real interviewing – Has it been a while since you last interviewed for a position? If you don’t interview for positions on a regular basis you can get a bit rusty. The best way to avoid this trap is to interview for positions regularly even if you’re happy with your current position. You never know, you may get an offer you can’t refuse.
Fundamental C# programming interview questions
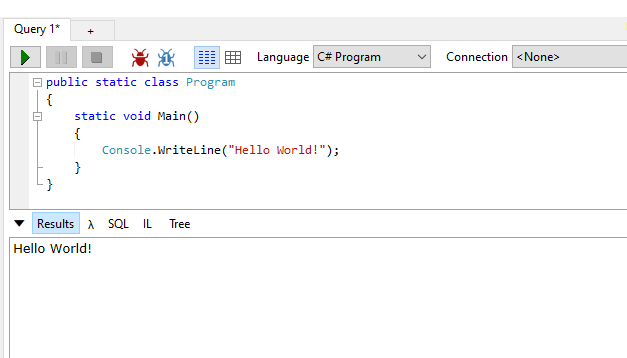
In any C# programming interview, you are bound to be thrown a few basic questions about the C# language. Any programmer will have good general knowledge about their language.
However, if you don’t get the answers 100% correct, don’t sweat because it is still possible to do well in your interview if you can demonstrate that you have a general understanding of the language at a high level.
Here are some basic C# programming interview questions that you could encounter during an interview.
What is C#?
C# is an object-oriented type-safe programming language developed by Microsoft for use on the .Net Framework to solve business problems. It is in the C family of languages like Java and Javascript.
What are some important features of C#?
- It is type-safe so that invalid operations cannot be performed on objects.
- Garbage collection that reclaims memory automatically.
- Exception handling allows for errors to be recovered from in a structured way.
- A unified type system so that all types inherit from the object type. This allows all types to share common operations.
What does the virtual keyword do?
The virtual keyword allows for methods and properties of a class to be overridden in classes that inherit from the class that uses the virtual keyword.
What is the difference between “throw” and “throw ex”?
“throw” allows you to throw an exception and indicate that an error has occurred while running a program. The exception object contains information about where the error occurred such as the Stack Trace. The Stack Trace provides details about which file and on what line the exception was thrown.
“throw ex” rethrows an Exception named ex that has been caught by a try-catch statement. The downside of doing this is that it resets information such as the Stack Trace so that the real starting point of the error is removed.
For more information about the intricacies of the C# programming language, you may want to study Microsoft’s guide to C#.
Tools to help you practice
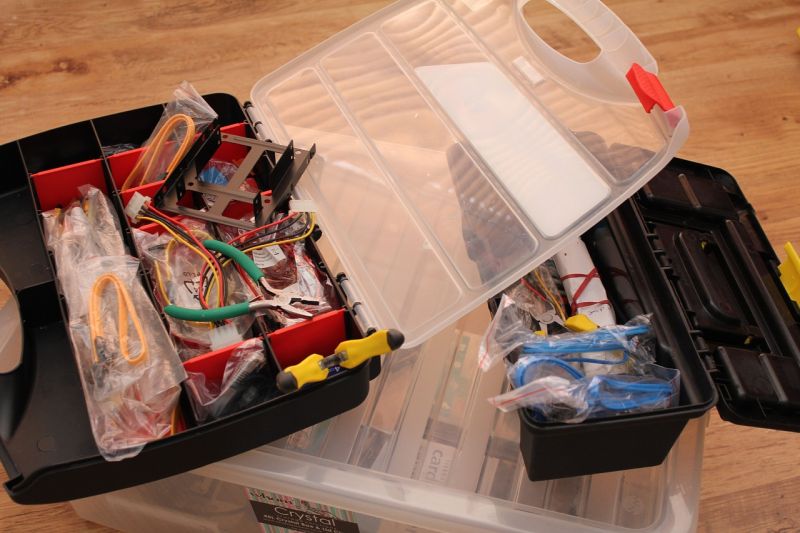
There are a number of tools that you can practice programming in C# while preparing for a programming interview.
If you don’t have your machine set up for .net development that is not a problem. In that case, give dotnetfiddle.net a try. It will allow you to solve quite a few C# programming practice problems without spending time setting up a full development environment.
If you’d prefer more of an offline solution but don’t want to spend too much time on setup then give linqpad.net a try. It is a great little scratch pad that is free with IntelliSense the only feature you need to pay for….and even then it is well worth the investment.
Now, if you’ve got a bit more time on your hands and want to practice using tools that professional C# developers use every day then you can’t go wrong with either Visual Studio Community Edition or in a pinch Visual Studio Code.
Sample C# programming problems to solve
There are dozens of sites online that will provide you with problems to practice solving. Take some time to work through as many as you can.
Here are a few problems that I would be happy to ask any aspiring developer.
FizzBuzz – Quickly weed out people who can’t code
Originally a children’s game, this is a simple programming problem that any C# programmer could solve in a few minutes. The intention behind asking it is to weed out anyone who cannot code rather than to test the depths of one’s programming ability.
- Write a program that prints the numbers 1 to 100
- If the number is a multiple of 3, print the word “Fizz”
- When the number is a multiple of 5, print the word “Buzz”
- For numbers that are a multiple of both 3 and 5, print the word “FizzBuzz”
There are many solutions to this problem. Here is my attempt at a solution.
private void FizzBuzz() { for (int i = 1; i <= 100; i++) { if (i % 3 == 0 && i % 5 == 0) { Console.WriteLine("FizzBuzz"); } else if (i % 3 == 0) { Console.WriteLine("Fizz"); } else if (i % 5 == 0) { Console.WriteLine("Buzz"); } else { Console.WriteLine(i); } } }
String Calculator – A great C# programming problem
There is a place for a more detailed assessment of a potential recruit’s skills in any set of C# programming interview questions. This can involve asking them to complete a programming task that can then be discussed at length.
The following problem is a coding kata created by Roy Osherove. It’s designed to be daily unit testing practice but also makes a wonderful interview question because it can be worked on in stages and all design choices discussed. A developer should be able to code a solution to this problem in 30 to 60 minutes.
Create a String Calculator by performing the following tasks in order and one at a time.
- Create a method called Add that takes a string of numbers and returns an int. (solution)
- The string of numbers will be a maximum of two numbers separated by commas
- The method will return their sum
Examples of input:
“” returns 0
“1” returns 1
“1,2” returns 3
- Enhance the method to take unlimited numbers (solution)
- Enhance the method to also handle newlines as a number separator (solution)
Examples of input:
“1\n2,3” returns 6
“1,\n” is invalid but you don’t need to handle this scenario.
- Enhance the method to allow custom delimiters (solution)
- The custom delimiter will be specified at the beginning of the string of numbers in a special format.
- The basic format of the string of numbers will be “//[delimiter]\n[numbers”
- The custom delimiter is optional. If not present, comma and newline will be the default delimiters.
Example of input:
“//;\n1;2” returns 3
- Throw an exception with message “negatives not allowed” plus all the negative numbers that were passed. (solution)
Example of input:
“1,2,-3,4,-5” results in exception with message “negatives not allowed -3, -5”
Here is a basic solution to this problem:
Task 1 Solution – Create Add method
Our first step in solving this C# programming puzzle is to create a minimal solution that addresses the stated criteria. This task allows an interviewer to see how a developer solves a problem without knowing the full specification. Good programming interview questions can bring out the pragmatic problem-solving skills of a developer.
In this solution, we don’t worry about edge cases but instead only implement what is required. Gold plating at this stage would be premature optimization.
public int Add(string numbers) { char delimiter = ','; if(string.IsNullOrWhiteSpace(numbers)) return 0; int delimiterIndex = numbers.IndexOf(delimiter); if (delimiterIndex < 0) { return int.Parse(numbers); } string firstNumber = numbers.Substring(0, delimiterIndex); string secondNumber = numbers.Substring(delimiterIndex+1, numbers.Length - firstNumber.Length - 1); return int.Parse(firstNumber) + int.Parse(secondNumber); }
Task 2 Solution – Allow unlimited numbers
This new requirement allows us to achieve a much more elegant solution to this problem. Instead of extracting the individual numbers ourselves, we can leverage the Split function.
public int Add(string numbers) { char delimiter = ','; if(string.IsNullOrWhiteSpace(numbers)) return 0; string[] values = numbers.Split(delimiter); int sum = 0; foreach (var value in values) { sum += int.Parse(value); } return sum; }
Task 3 Solution – Support Newline delimiter
Supporting a newline character requires us to refactor our original solution of representing our delimiter as a single character. Being able to refactor code when new information becomes available to us is a great way to test a developer’s real-world problem-solving skills in a C# programming interview question.
public int Add(string numbers) { string[] delimiters = new string[] { ",", "\n"}; if(string.IsNullOrWhiteSpace(numbers)) return 0; string[] values = numbers.Split(delimiters, StringSplitOptions.None); int sum = 0; foreach (var value in values) { sum += int.Parse(value); } return sum; }
Task 4 Solution – Support custom delimiter
Here we have solved the next stage of this programming puzzle by adding in support for custom delimiters.
We do this by identifing and extracting the delimiter before removing it so the remainder of the numbers can be processed as before. One could also consider refactoring this addition to a separate method.
public int Add(string numbers) { List<string> delimiters = new List<string> { ",", "\n"}; if(string.IsNullOrWhiteSpace(numbers)) return 0; if (numbers.StartsWith("//")) { int startDelimiterIndex = 2; int endDelimiterIndex = numbers.IndexOf("\n")+1; int delimiterLength = endDelimiterIndex - (startDelimiterIndex + 1); delimiters.Add(numbers.Substring(startDelimiterIndex, delimiterLength)); //remove the delimiter specifier formatting from the numbers input numbers = numbers.Substring(endDelimiterIndex, numbers.Length - endDelimiterIndex); } string[] values = numbers.Split(delimiters.ToArray(), StringSplitOptions.None); int sum = 0; foreach (var value in values) { sum += int.Parse(value); } return sum; }
Task 5 Solution – Exception for negatives
In this solution, we keep a list of negative values. If there are any values present, we can include them in the Exception message.
This final solution is only one possible solution for this C# programming problem. It is not intended to be the definitive or most elegant solution. Instead, this solution solves the problem in a way that should be readily achievable during a programming interview.
One way to make the code more maintainable would be to refactor the method to better comply with the Single Responsibility Principle.
public int Add(string numbers) { List<string> delimiters = new List<string> { ",", "\n"}; if(string.IsNullOrWhiteSpace(numbers)) return 0; if (numbers.StartsWith("//")) { int startDelimiterIndex = 2; int endDelimiterIndex = numbers.IndexOf("\n")+1; int delimiterLength = endDelimiterIndex - (startDelimiterIndex + 1); delimiters.Add(numbers.Substring(startDelimiterIndex, delimiterLength)); //remove the delimiter specifier formatting from the numbers input numbers = numbers.Substring(endDelimiterIndex, numbers.Length - endDelimiterIndex); } string[] values = numbers.Split(delimiters.ToArray(), StringSplitOptions.None); int sum = 0; List<int> negativeValues = new List<int>(); foreach (var value in values) { int parsedValue = int.Parse(value); sum += parsedValue; if (parsedValue < 0) negativeValues.Add(parsedValue); } if (negativeValues.Count > 0) { throw new Exception($"negatives not allowed {String.Join(",", negativeValues)}"); } return sum; }
What else can you do to prepare for C# programming interview questions?
Now that you’ve taken the time to prepare for your next C# programming interview, what else can you do?
Take action!
At the end of the day, preparation will only get you so far. The only way to get that job is to take action and go through the interview process.
Sure, you might make mistakes…and possibly fail a few interviews…but studying C# programming problems forever also won’t land you that dream job.
If you’re still struggling with your confidence, one thing that can help is practical experience solving real-world business problems with C#. A good way to build that experience can be by volunteering your time on open source software projects. Solving real-world problems is way better than studying practice problems and C# programming reference manuals.
One Comment
Comments are closed.